Table of Contents
- Compiling and degubbing code for Tiva
- Hardware Abstraction Layer
- Debugging, heap, and display
- Analog Digital Converter and Timers
- Playing Nokia Tunes
- Random Number Generator, Rendering Engine, and the Game
- A real-time operating system
DAC
Tiva does not have a DAC, but we'd like to have some sound effects while playing the game. Fortunately, it's easy to make a simple binary-weighted DAC using resistors and GPIO signals. It's not very accurate, but will do.
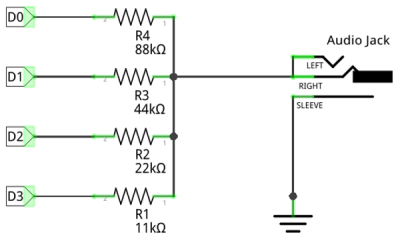
As far as the software is concerned, we will simply take 4 GPIO pins and set them up as output. We will then get an appropriate bit-banded alias such that writing an integer to it is reflected only in the state of these four pins.
1int32_t IO_dac_init(IO_io *io, uint8_t module)
2{
3 if(module > 0)
4 return -IO_EINVAL;
5
6 TM4C_gpio_port_init(GPIO_PORTD_NUM);
7 TM4C_gpio_pin_init(GPIO_PORTD_NUM, GPIO_PIN0_NUM, 0, 0, 1);
8 TM4C_gpio_pin_init(GPIO_PORTD_NUM, GPIO_PIN1_NUM, 0, 0, 1);
9 TM4C_gpio_pin_init(GPIO_PORTD_NUM, GPIO_PIN2_NUM, 0, 0, 1);
10 TM4C_gpio_pin_init(GPIO_PORTD_NUM, GPIO_PIN3_NUM, 0, 0, 1);
11
12 uint32_t addr = GPIO_REG_BASE + GPIO_PORTD;
13 addr += GPIO_PIN0_BIT_OFFSET;
14 addr += GPIO_PIN1_BIT_OFFSET;
15 addr += GPIO_PIN2_BIT_OFFSET;
16 addr += GPIO_PIN3_BIT_OFFSET;
17 dac_data = (uint32_t*)addr;
18
19 io->type = IO_DAC;
20 io->sync = 0;
21 io->channel = 0;
22 io->flags = 0;
23 io->read = 0;
24 io->write = dac_write;
25 io->event = 0;
26
27 return 0;
28}
See TM4C_platform01.c.
Sound
We will create a virtual device consisting of a DAC and a timer. Using the timer, we will change the output of the DAC frequently enough to produce sound. Since the timer interrupt needs to be executed often and any delay makes the sound break, we need to assign the highest possible priority to this interrupt so that it does not get preempted.
1int32_t IO_sound_init(IO_io *io, uint8_t module)
2{
3//...
4 IO_dac_init(&snd_dac, 0);
5 IO_timer_init(&snd_timer, 11);
6 TM4C_enable_interrupt(104, 0); // adjust the interrupt priority for timer 11
7 snd_timer.event = snd_timer_event;
8//...
9}
Writing to this virtual device sets the frequency of the tone that we want to play by adjusting the timer's firing rate accordingly.
1static int32_t snd_write(IO_io *io, const void *data, uint32_t length)
2{
3 if(length != 1)
4 return -IO_EINVAL;
5 const uint64_t *val = data;
6
7 if(!(*val)) {
8 snd_interval = 0;
9 return 1;
10 }
11 uint8_t turn_on = 0;
12 if(!snd_interval)
13 turn_on = 1;
14
15 double interval = 1.0/(*val);
16 interval /= 32.0;
17 interval *= 1000000000;
18 snd_interval = interval;
19
20 if(turn_on)
21 IO_set(&snd_timer, interval);
22 return 1;
23}
In reality, the timer fires 32 times more often than the frequency of the tone requires. It is because we use a table with 32 entries to simulate the actual sound wave. In principle, we could just use a sinusoid, but it turns out that the quality of the sound is not so great if we do so. I have found another waveform in the lab materials of EdX's Embedded Systems course that works much better.
1static const uint8_t snd_trumpet[] = {
2 10, 11, 11, 12, 10, 8, 3, 1, 8, 15, 15, 11, 10, 10, 11, 10, 10, 10, 10,
3 10, 10, 10, 10, 11, 11, 11, 11, 11, 11, 10, 10, 10 };
4
5static void snd_timer_event(IO_io *io, uint16_t event)
6{
7 IO_set(&snd_dac, snd_trumpet[snd_step++]);
8 snd_step %= 32;
9 if(snd_interval)
10 IO_set(io, snd_interval);
11}
See TM4C_platform01.c.
Nokia tunes
The tune player API consists of four functions:
1int32_t IO_sound_play(IO_io *io, IO_io *timer, IO_tune *tune, uint16_t start);
2int32_t IO_sound_stop(IO_io *io);
3IO_tune *IO_sound_decode_RTTTL(const char *tune);
4void IO_sound_free_tune(IO_tune *tune);
IO_sound_play
uses a sound device and a timer to play a tune. It sends anIO_EVENT_DONE
to the virtual sound device when the playback finishes.IO_sound_stop
stops the playback on the given device and returns the index of the last note it played so that it can be restarted from that point.IO_sound_decode_RTTL
takes an RTTTL representation and produces theIO_tune
structure that can be handled by the player.IO_sound_free_tune
frees the memory used byIO_sound_decode_RTTL
when it's no longer needed.
There is plenty of tunes all over the Internet. The ones in the demo video are taken from here. The code of the player is based on this work.
See IO_sound.c.
If you like this kind of content, you can subscribe to my newsletter, follow me on Twitter, or subscribe to my RSS channel.